To remove an object in KineticJS, you can use the remove() method on the object you want to delete. This method will remove the object from the KineticJS stage, but it will not delete the object from memory. If you want to completely delete the object, you can use the destroy() method instead. This will remove the object from the stage and delete it from memory. Remember to call the draw() method on the stage after removing or destroying an object to see the changes reflected on the canvas.
Best Javascript Books to Read in November 2024
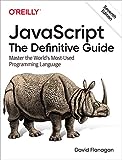
Rating is 5 out of 5
JavaScript: The Definitive Guide: Master the World's Most-Used Programming Language
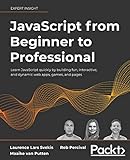
Rating is 4.9 out of 5
JavaScript from Beginner to Professional: Learn JavaScript quickly by building fun, interactive, and dynamic web apps, games, and pages
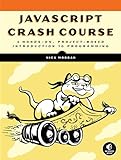
Rating is 4.8 out of 5
JavaScript Crash Course: A Hands-On, Project-Based Introduction to Programming
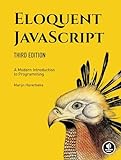
Rating is 4.7 out of 5
Eloquent JavaScript, 3rd Edition: A Modern Introduction to Programming
- It can be a gift option
- Comes with secure packaging
- It is made up of premium quality material.
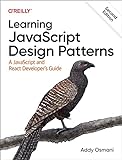
Rating is 4.5 out of 5
Learning JavaScript Design Patterns: A JavaScript and React Developer's Guide
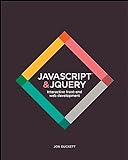
Rating is 4.4 out of 5
JavaScript and jQuery: Interactive Front-End Web Development
- JavaScript Jquery
- Introduces core programming concepts in JavaScript and jQuery
- Uses clear descriptions, inspiring examples, and easy-to-follow diagrams
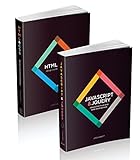
Rating is 4.3 out of 5
Web Design with HTML, CSS, JavaScript and jQuery Set
- Brand: Wiley
- Set of 2 Volumes
- A handy two-book set that uniquely combines related technologies Highly visual format and accessible language makes these books highly effective learning tools Perfect for beginning web designers and front-end developers
What is the consequence of not removing objects in KineticJS?
Not removing objects in KineticJS can lead to memory leaks and decreased performance of the application. As objects continue to accumulate in the stage, they consume more memory and processing power, slowing down the rendering and execution of the application. This can result in lag, decreased responsiveness, and potential crashes. Additionally, it can also lead to issues with managing resources and potentially cause unexpected behaviors in the application. It is important to properly remove objects from the stage in order to maintain optimal performance and efficiency.
What is the best practice for removing objects in KineticJS?
The best practice for removing objects in KineticJS is to use the remove() method. This method removes the specified node from the layer or group it belongs to. Here is an example of how to remove an object in KineticJS:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
// Create a KineticJS stage var stage = new Kinetic.Stage({ container: 'container', width: 500, height: 500 }); // Create a KineticJS layer var layer = new Kinetic.Layer(); // Create a KineticJS rectangle var rect = new Kinetic.Rect({ x: 100, y: 100, width: 50, height: 50, fill: 'blue' }); // Add the rectangle to the layer layer.add(rect); // Add the layer to the stage stage.add(layer); // Remove the rectangle from the layer rect.remove(); // Draw the stage stage.draw(); |
In this example, the remove()
method is called on the rect
object to remove it from the layer. This is a simple and effective way to remove objects in KineticJS.
How to remove a specific shape in KineticJS?
To remove a specific shape in KineticJS, you can use the following steps:
- Identify the shape you want to remove. You can do this by giving the shape a unique ID or name when you create it.
- Use the find() method to get a reference to the specific shape you want to remove. For example, if you gave the shape an ID of "myShape", you can use find("#myShape") to get a reference to it.
- Once you have a reference to the shape, you can use the destroy() method to remove it from the stage. For example:
1 2 3 |
var shape = layer.find("#myShape")[0]; // Get a reference to the shape shape.destroy(); // Remove the shape from the stage layer.draw(); // Redraw the layer |
By following these steps, you can easily remove a specific shape in KineticJS.