To change a number to a negative number in Kotlin, you can simply multiply the number by -1. For example, if you have a variable num
with a value of 5 and you want to make it -5, you can do so by writing num = num * -1
. This will change the number to its negative equivalent.
Best Kotlin Books to Read in 2024
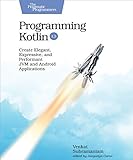
Rating is 4.7 out of 5
Programming Kotlin: Create Elegant, Expressive, and Performant JVM and Android Applications
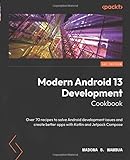
Rating is 4.6 out of 5
Modern Android 13 Development Cookbook: Over 70 recipes to solve Android development issues and create better apps with Kotlin and Jetpack Compose
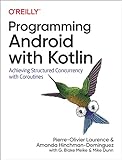
Rating is 4.4 out of 5
Programming Android with Kotlin: Achieving Structured Concurrency with Coroutines
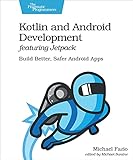
Rating is 4.3 out of 5
Kotlin and Android Development featuring Jetpack: Build Better, Safer Android Apps
How to utilize Kotlin's built-in functionality to change a number to negative?
You can utilize Kotlin's built-in functionality by using the unaryMinus()
operator to change a number to negative. Here's an example code snippet:
1 2 3 4 5 |
val number = 10 val negativeNumber = -number // using unaryMinus() operator println("Original Number: $number") // Output: 10 println("Negative Number: $negativeNumber") // Output: -10 |
In this example, the unaryMinus()
operator is used to change the number 10
to its negative counterpart -10
.
What is the recommended approach for changing a number to negative in Kotlin?
One recommended approach for changing a number to negative in Kotlin is to multiply it by -1. Here is an example:
1 2 3 |
val number = 5 val negativeNumber = number * -1 println(negativeNumber) // Output: -5 |
This method is simple and efficient for changing a number to negative in Kotlin.
What is the syntax for changing a number to a negative number in Kotlin?
To change a number to a negative number in Kotlin, you can simply multiply the number by -1. Here is an example:
1 2 3 4 |
val number = 5 val negativeNumber = number * -1 println(negativeNumber) // Output: -5 |